Guarding Your Firebase Wallet: Defending Against Denial of Wallet Attacks
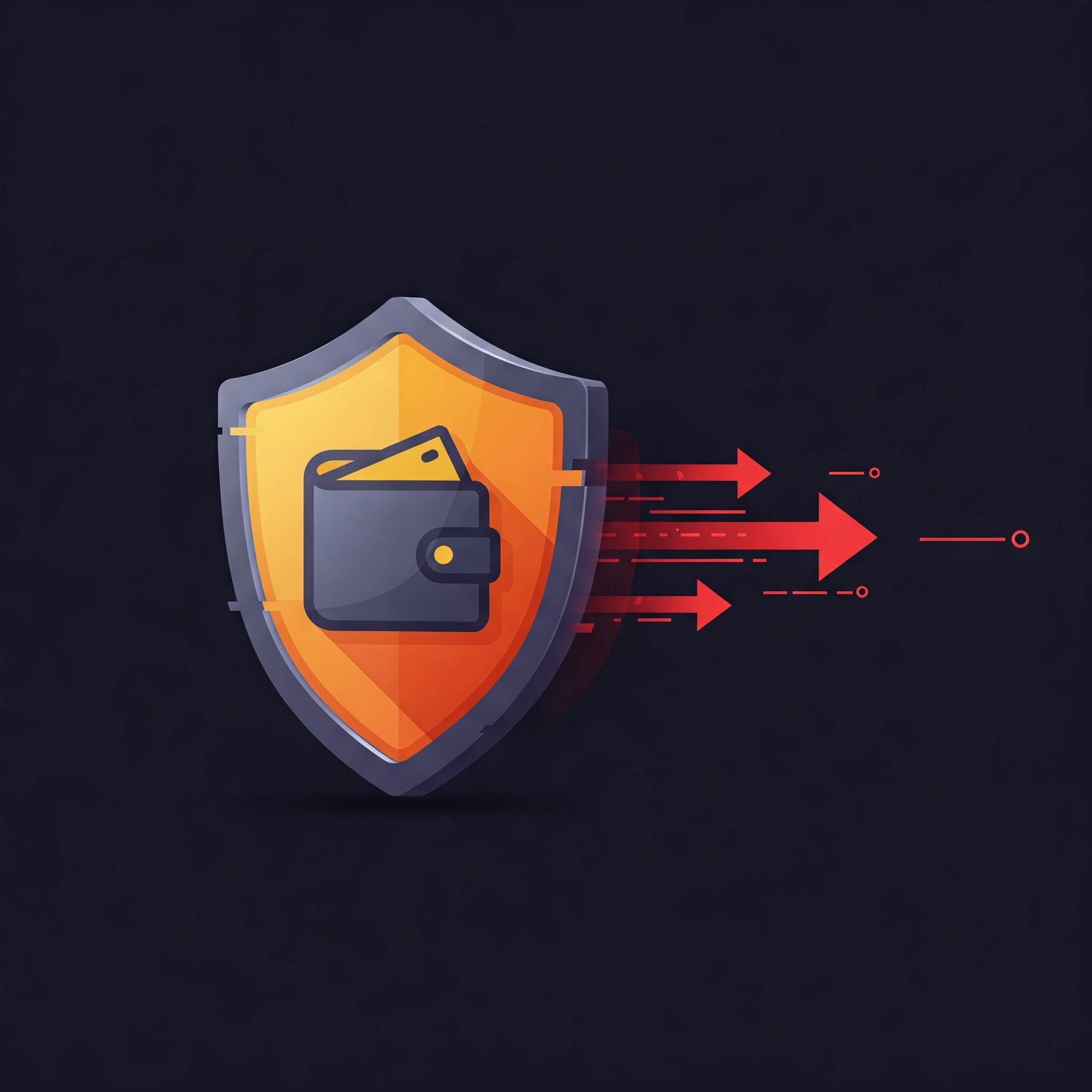
The Bandits of the Blaze Plan Are About to Target Firebase
Developers often face unexpected Firebase bills due to rogue queries or overlooked function calls. It’s a common headache. But a more sinister threat is emerging: the Denial of Wallet (DoW) attack. Instead of simply disrupting your service, attackers are now targeting your cloud budget, aiming to drain your resources through inflated usage costs.
This post explores how DoW attacks could become a significant problem in the Firebase ecosystem, illustrates the potential financial damage with examples, explains why this threat is growing, and—most importantly—details how to implement robust defenses for your projects before it’s too late. We’ll cover Firebase security best practices and introduce tools designed for this specific challenge.
Understanding Denial of Wallet Attacks on Firebase
A Denial of Wallet (DoW) attack is a malicious act focused on financial sabotage. Unlike traditional Denial of Service (DoS) attacks that aim to take systems offline, a DoW attack weaponizes usage-based pricing models to inflict financial damage.
Firebase’s flexible pay-as-you-go pricing, while powerful for scaling, presents a vulnerability. Attackers can deliberately trigger billable events excessively, artificially inflating usage and leaving you with a potentially massive bill. Their goal isn’t just disruption; it’s to exhaust your budget.
How DoW Attacks Could Target Firebase
DoW attacks primarily exploit Firebase services with cost-per-use billing. Here’s a breakdown of common vectors:
- Firebase Functions: Attackers can flood HTTP-triggered Cloud Functions with millions of requests. Even fractions of a penny per invocation add up alarmingly fast at scale, especially for functions performing moderately complex tasks.
- Cloud Storage: Malicious actors might upload or download huge volumes of data, driving up storage and, more significantly, egress fees. Publicly accessible or poorly secured buckets are prime targets.
- Firestore: Generating fake user activity to spam database reads or writes can lead to substantial bills. Complex queries or writes that trigger downstream Cloud Functions can amplify these costs significantly.
- Gemini-Enabled Functions / AI Services: Repeatedly invoking expensive generative AI models or other specialized AI features, particularly those involving large inputs like media files, can rapidly escalate costs.
Two Main Attack Patterns
DoW attacks often manifest in two primary ways:
- Burst Attack: This involves flooding Firebase services with an overwhelming volume of requests in a short period, causing a rapid cost spike. Example: Triggering an image-processing Cloud Function thousands of times per minute.
- Leech Attack: This is a slower, more insidious approach. Attackers mimic seemingly legitimate, high-usage user behavior over an extended period. Example: Creating numerous fake accounts performing frequent Firestore reads/writes that stay just below obvious abuse thresholds.
Illustrative Examples: The Cost of a DoW Attack
The financial consequences of a DoW attack can range from annoying to catastrophic. Here are a few simplified, illustrative examples (Note: Actual costs depend on specific configurations, regions, and current pricing; these use common estimates for demonstration):
- Scenario 1: Cloud Function Burst Attack
- Attack: An attacker triggers a simple, unsecured HTTP Cloud Function 20 million times over 24 hours. Assume the function runs quickly (minimal compute time cost for this example).
- Illustrative Cost: Beyond the free tier (typically ~2 million invocations/month), the cost might be around $0.40 per million invocations. This attack could generate roughly (18 million / 1 million) * $0.40 = ~$7.20 in invocation costs alone, plus compute time charges which could add significantly more depending on function complexity and duration. Scaling this to hundreds of millions of requests is trivial for attackers.
- Scenario 2: Firestore Read Leech Attack
- Attack: An attacker uses bots to simulate 5,000 fake users, each performing 2,000 document reads per day from a Firestore collection for 5 days.
- Illustrative Cost: This totals 5,000 users * 2,000 reads/user/day * 5 days = 50 million reads. After the daily free tier (e.g., 50,000 reads/day), costs might be around $0.06 per 100,000 reads. Ignoring the free tier for simplicity in this large attack, the cost could be roughly (50,000,000 / 100,000) * $0.06 = ~$30. While seemingly small initially, this type of attack can run undetected for longer periods, accumulating costs steadily.
- Scenario 3: Cloud Storage Egress Attack
- Attack: An attacker repeatedly downloads a large, publicly accessible 250MB video file from Cloud Storage, generating 2 Terabytes (TB) of egress traffic.
- Illustrative Cost: After the monthly free tier (e.g., 10 GB), egress costs might be around $0.12 per GB. This attack could generate costs of approximately (2048 GB - 10 GB free) * $0.12/GB = ~$244 in egress fees.
- Scenario 4: AI Function Abuse
- Attack: An attacker finds an unsecured function that uses a specialized AI model (e.g., complex image analysis, generative text) and triggers it 500,000 times.
- Illustrative Cost: AI function calls can be significantly more expensive than standard functions. Even a hypothetical $0.01 per call (which could be low for complex models) would result in 500,000 * $0.01 = ~$5,000. Costs for advanced AI services can escalate extremely rapidly.
These examples highlight how automated attacks targeting usage-based services can quickly lead to substantial, unexpected bills.
Why DoW Attacks on Firebase Are a Growing Threat
Several converging factors make Firebase an increasingly attractive target for DoW attacks:
- Wider Adoption: Firebase’s popularity means more potential targets.
- Improved DDoS Defences: As traditional DDoS mitigation improves, attackers pivot to alternative strategies like cost-based attacks.
- Rising Costs of Advanced Features: Powerful (and expensive) features like AI integrations increase the potential financial damage per malicious request.
- Architectural Vulnerabilities: Firebase services are often managed distinctly (Firestore, Storage, Functions), and native Function rate limiting can be less granular than dedicated API gateways, potentially increasing the attack surface.
- Billing Limitations: Firebase and Google Cloud often require manual intervention to halt escalating costs, even after budget alerts trigger. Damage can accrue before action is taken.
- Motivated Actors: Motivations range from corporate sabotage and extortion demands to sheer vandalism.
Why Standard Defenses Can Be Difficult
Stopping DoW attacks is challenging because:
- They often mimic legitimate user traffic, making detection hard.
- They exploit the very auto-scaling and responsiveness features that make Firebase attractive.
- Firebase/Google Cloud’s real-time cost monitoring and alerting can have delays, allowing significant costs to accumulate before you’re notified.
Protecting Your Firebase Projects: A Multi-Layered Approach
Defending against DoW requires a combination of Firebase features and potentially specialized tooling. Here’s how to bolster your defenses:
1. Use Firebase App Check
Ensure only verified instances of your app can access your backend services. This adds significant friction for unsophisticated automated attacks but isn’t foolproof against determined attackers.
2. Implement Robust Security Rules
Apply the principle of least privilege rigorously. Define granular access controls for Firestore, Realtime Database, and Cloud Storage.
Example Firestore Rule (restricting writes to authenticated owners):service cloud.firestore {
match /databases/{database}/documents/{document=**} {
// Allow writes only if the user is authenticated and their UID matches the document's uid field
allow write: if request.auth != null && request.auth.uid == resource.data.uid;
// Allow reads more broadly (adjust as needed)
allow read: if true;
}
}
You can also implement basic time-based write limiting directly in rules, like this example which prevents updates within 5 seconds of the previous one, useful for mitigating rapid-fire abuse on specific documents:
match /users/{document=**} {
allow create: if isMine() && hasTimestamp();
allow update: if isMine() && hasTimestamp() && isCalm();
function isMine() {
// Ensure the user is updating their own document
return request.auth.uid == document;
}
function hasTimestamp() {
// Ensure the incoming data includes a server timestamp
return request.resource.data.timestamp == request.time;
}
function isCalm() {
// Allow update only if 5 seconds have passed since the last update
// Assumes the document being updated (`resource`) has a 'timestamp' field from the previous write
return request.time > resource.data.timestamp + duration.value(5, 's');
}
}
While helpful, implementing complex rate limiting across many users or resources directly in security rules can become difficult to manage and may not cover all attack vectors, especially those targeting Cloud Functions or Storage.
3. Implement Effective Rate Limiting and Throttling
Preventing excessive function executions, logins, and database operations is crucial. While you can build basic custom rate limiting yourself (as partly shown in the security rules example above, or using middleware in Cloud Functions), these approaches often have limitations against sophisticated attacks:
- Basic IP-based limiting: Can be easily bypassed using proxies or botnets and may inadvertently block legitimate users behind shared IPs.
- Complexity: Implementing robust, user-aware, or resource-specific rate limiting manually adds significant development overhead.
- Maintenance: Keeping custom logic up-to-date with evolving attack vectors requires ongoing effort.
For robust, Firebase-specific protection without the maintenance headache, consider a dedicated solution. Flame Shield (flamesshield.com) provides advanced rate limiting and throttling capabilities specifically designed to integrate easily and protect Firebase projects from DoW attacks and other abuse patterns. It offers more granular control than basic IP limiting or simple rule-based timing, helping you block bad actors without impacting legitimate users.
For more information on advanced rate limiting strategies, see Flame Shield’s DDoS protection features.
4. Employ a Kill Switch
Beyond rate limiting, a kill switch is an indispensable emergency brake. In a severe DoW attack scenario where costs are rapidly escalating, you need the ability to instantly halt all traffic to your Firebase project to stop the financial bleeding.
Manually disabling services can be slow and cumbersome during a crisis. Flame Shield (flamesshield.com) includes a kill switch feature, allowing you to cut off all Firebase traffic immediately with a single action, providing critical real-time mitigation against devastating cost overruns. This capability offers peace of mind that basic Firebase controls alone cannot provide.
5. Set Sensible Scaling Limits
Configure maximum instance counts for Cloud Functions in the Google Cloud Console. While this doesn’t prevent attacks, it can cap the maximum potential cost from function invocations during a single attack wave.
6. Monitor Billing and Usage Vigilantly
- Set up Google Cloud Budgets: Configure alerts for when spending approaches or exceeds defined thresholds. Use programmatic notifications via Pub/Sub for automated responses if feasible (You can find the notification format details here: https://cloud.google.com/billing/docs/how-to/budgets-programmatic-notifications#notification_format).
- Use Firebase Dashboards: Regularly check the built-in usage dashboards for anomalies.
- Consider Anomaly Detection: Explore tools that can identify unusual patterns potentially indicative of an attack. Remember that alerts often have delays.
7. Audit Regularly
Periodically review security rules, access controls, function configurations, and usage patterns to proactively identify and close potential vulnerabilities.
8. Leverage the Local Emulator Suite
Develop and test locally whenever possible using the Firebase Local Emulator Suite to avoid incurring unnecessary cloud costs and potentially exposing unfinished features during development.
Budget Defence Is Security Too
Denial of Wallet attacks, though perhaps less sensationalized than ransomware, can be equally devastating to projects and businesses, as the examples illustrate. In the cloud-native world, robust financial protection is a critical component of your overall security posture.
Firebase offers essential tools, including security rules for basic rate limiting, but shielding your project from sophisticated DoW attacks often requires dedicated defenses. Solutions like Flame Shield (flamesshield.com) provide the specialized rate limiting, throttling, and crucial kill switch functionality needed to effectively safeguard your Firebase budget against this growing threat.
Ready to secure your Firebase project against costly DoW attacks? Learn how Flame Shield offers tailored protection at flamesshield.com.